You can get machine translation and translation quality scores from the /translate
endpoint. This endpoint/class takes three parameters:
text
- The text to translate.source_lang
- The ISO 639 source language code (e.g. ‘eng’ for English).target_lang
- The ISO 639 target language code (e.g ‘fra’ for French).
Under the hood, the /translate
endpoint simultaneously leverages multiple state-of-the-art LLM and machine translation models to perform translations. The translations from these models are scored and ranked using reference-free quality estimation models. This allows us to select the highest quality machine translation for the given source and target languages.
Supported Languages
Our translation API supports a wide range of languages, including but not limited to English, Hindi, French, Spanish, German, and more. Refer to the language codes to identify specific languages.
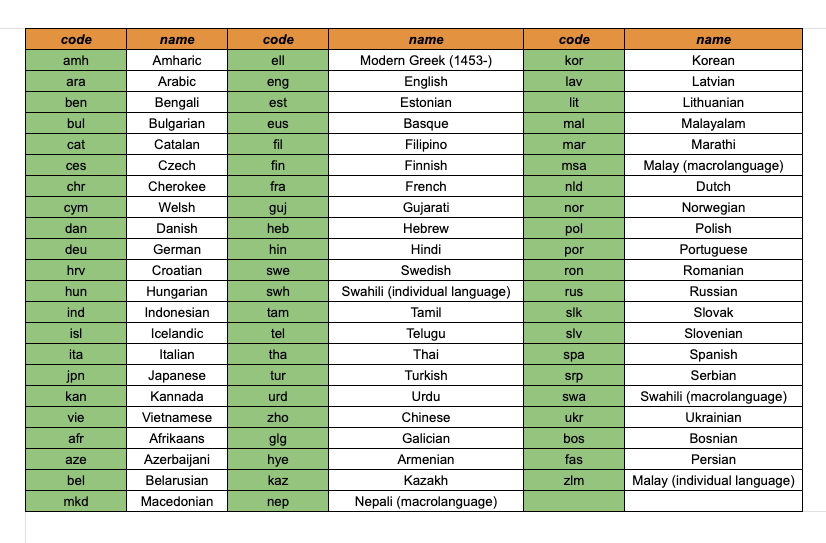
/translate
The /translate endpoint will return a JSON object response with two fields:
Best translation
- The translated text.Score
- A score from -1.0 to 1.0 representing the translation quality. Higher the score better the quality.
Generate a translation
To generate a translation, you can use the following code examples. Depending on your preference or requirements, select the appropriate method for your application.
The output will look something like: